Media
Media
is a component that displays <img>
tags. It has a default empty alt
tag which is required for non-decorative images. If the image is descriptive, for example, when there is no complementary text, then a descriptive alt
tag must be used. The Media
component also utilizes the loading="lazy"
tag to optimize browser performance.
Accessibility features
- Sets a default empty
alt
tag, which is required for non-descriptive images
Usage
Accessible Astro exports one media-related components:
Media
: The image Component with a defaultalt
tag
Props
Media
Props
alt
- Description: Descriptive text to use as the
alt
attribute of the image - Type:
string
- Default:
''
- Description: Descriptive text to use as the
class
- Description: One or more class names, as a string, to be applied to the
img
tag - Type:
string
- Default:
undefined
- Description: One or more class names, as a string, to be applied to the
src
- Description: The source URL of the image
- Type:
string
- Default:
https://fakeimg.pl/640x360
Example
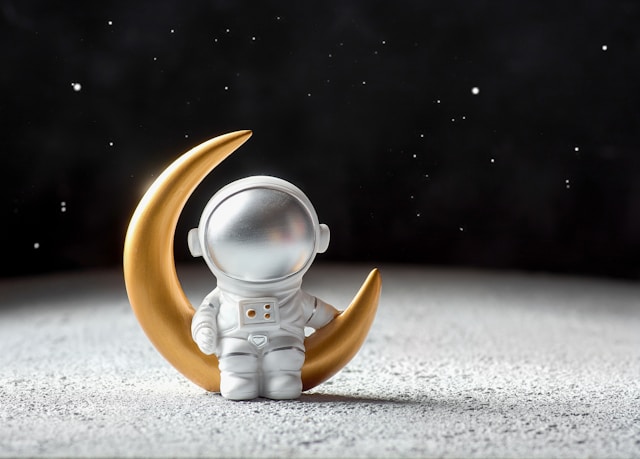
Photo by Kobby Mendez on Unsplash
---import { Media } from 'accessible-astro-components'---<Media classes="elevation-300 radius-large" src="https://unsplash.com/photos/d0oYF8hm4GI" alt="A tiny toy astronaut, sitting on a yellow toy moon"/>
Style customizations
The Media
component does not have any unique classes. Style it like other img
tags.